Session Replay
Prerequisites
Section titled PrerequisitesScreen tracking implemented
Section titled Screen tracking implementedAs session collection initiate upon the 1st screenview event, it’s essential to have screen tracking implemented. Make sure to follow the Flutter Track screens section.
Updating to latest SDK version
Section titled Updating to latest SDK versionTo enable Session Replay in your application and ensure optimal stability, make sure to always use the last SDK version.
Reminder about User consent
Section titled Reminder about User consentIf you are in the process of implementing the SDK for the 1st time, or if you choose to take this update as an opportunity to review your privacy-related implementation, ensure that you follow the Flutter Privacy section and use the Opt-in API to get the user consent, otherwise no data will be collected.
WebView
Section titled WebViewWebView events can be collected as part Session Replay under the following conditions:
- The web page implements the Web Tracking Tag.
- Your webview is wrapped with the
ContentsquareWebViewTrackerBuilder
widget - Your webview allows JavaScript execution
- The
ContentsquareWebViewTracker
methods are registered in the webview
For the full guide to implementation of Webview Tracking, see 📚 Mobile Apps Webview Tracking.
Testing and Debugging
Section titled Testing and DebuggingEnable Session Replay on your device
Section titled Enable Session Replay on your deviceSince not all sessions are collected (depending on the percentage set in the Contentsquare back office), we have implemented an option to force replay collection on your device for testing and debugging purposes. This option can be enabled from the in-app features settings:
- Enable in-app features
- Open in-app features settings with a long press on the snapshot button
- Under “Session Replay”, toggle “Enable Session Replay” on
- Kill the app
- Upon restarting the app, a new session will start with Session Replay enabled.
iOS example
Section titled iOS example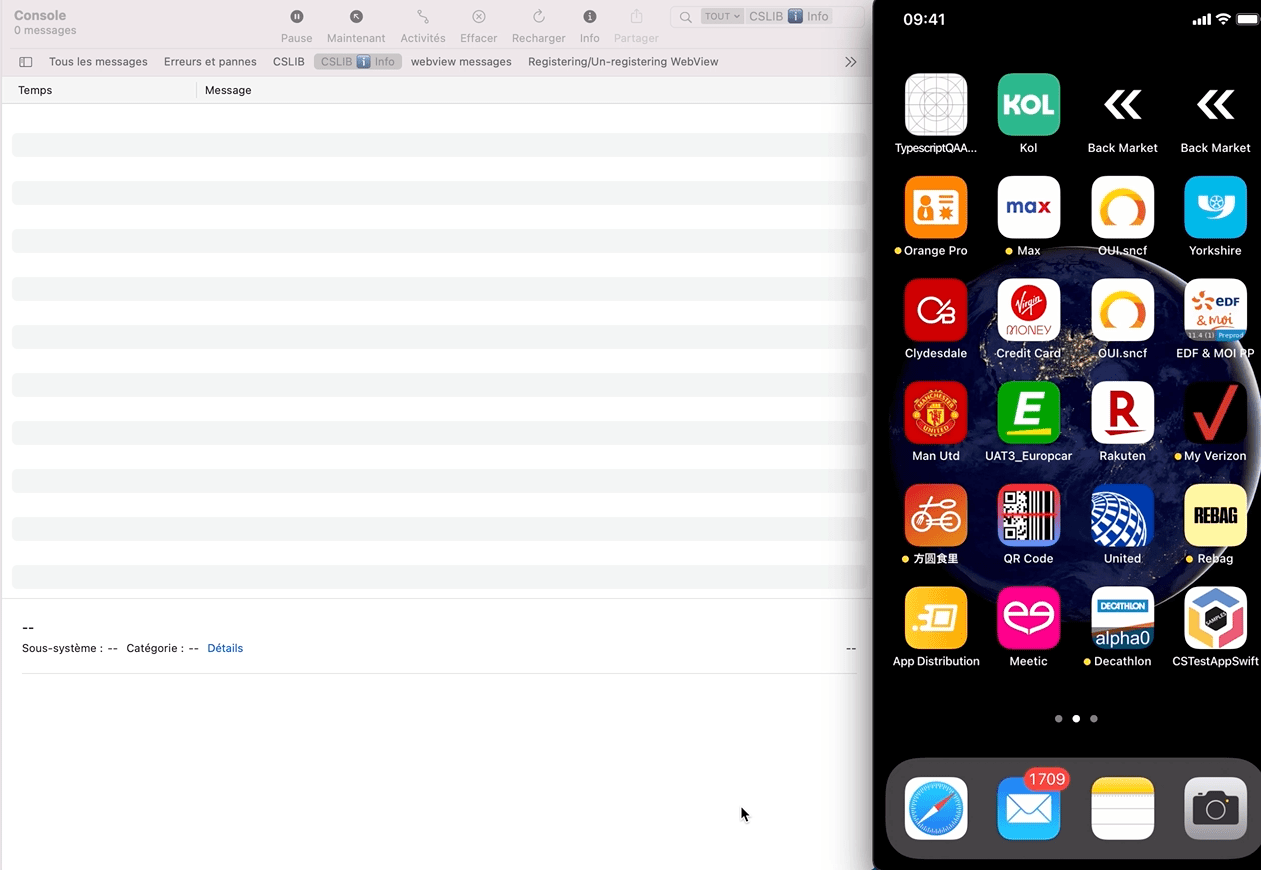
How do I know if Session Replay is enabled?
Section titled How do I know if Session Replay is enabled?There are 2 places where you can verify if Session Replay is enabled:
In the logs: The log Session Recording is starting
will confirm that Session Replay is enabled.
In in-app features settings: Below the “Enable “will start at next app start” (see below Access the replay), you will either see:
No replay link available
which means Session Replay is not running for the current sessionGet Replay link
which means Session Replay is running for the current session
Access the Replay
Section titled Access the ReplayThe session can be accessed by tapping on Get replay link
button from the in-app features settings:
The replay will be available within 5 minutes. Only the concluded screen views are processed, and we identify a screenview has concluded when we begin receiving data for the subsequent screenview or if the session has ended. This means that you will be able to replay your session up to the previous screenview if the session is still ongoing.
Get the replay link programmatically
Section titled Get the replay link programmaticallyThe onSessionReplayLinkChange
method is designed to provide a mechanism for tracking changes to the Session Replay URL in real-time.
Function signature
Section titled Function signaturevoid onSessionReplayLinkChange(void Function(Uri url)? onLinkChanged);
Parameters
Section titled ParametersonLinkChanged (void Function(Uri url)?)
: A callback function that receives the updated Session Replay URL. This parameter is nullable.
Key Features:
Section titled Key Features:-
Real-Time Updates: The callback is triggered every time there is a change in the Session Replay URL. It is also triggered when the callback is first registered, providing the current Session Replay URL.
-
Single Callback Registration: Only one callback can be active at a time. If the API is called multiple times, the most recent callback registration overrides the previous one.
-
Optional Callback: The
onLinkChanged
argument is nullable, allowing the API to be used to stop listening to URL changes.
Usage
Section titled UsageRegistering a Callback
Section titled Registering a CallbackTo start listening to changes:
Contentsquare().onSessionReplayLinkChange((Uri url) { /// Handle the updated Session Replay URL print('Session Replay URL: $url');});
Stopping the Listener
Section titled Stopping the ListenerTo stop listening to changes:
Contentsquare().onSessionReplayLinkChange(null);
Personal Data Masking
Section titled Personal Data MaskingThe Session Replay feature collects every user interaction within your app. In order to respect the user’s right to privacy, the Contentsquare SDK:
- Masks potentially user sensitive data by default (see Default masking)
- Provides APIs for controlling which parts of the user interface are collected through masking and unmasking functionalities.
Masking mechanisms
Section titled Masking mechanismsMasking depends on the type of element:
- Images: Not collected, instead a placeholder is sent in place of the content, and the “IMG” placeholder is displayed within the element’s frame.
- SVG images: Not collected, instead a placeholder is sent in place of the content, and the “IMG” placeholder is displayed within the element’s frame.
- CustomPaint: custom-made
CustomPaint
are not collected, instead a placeholder is sent in place of the content, and the “IMG” placeholder is displayed within the element’s frame. Framework specific decorativeCustomPaint
not containing any sensitive data are collected. - Text: Replaced by “la” repeated as many times as needed to equal the original character count. White characters are preserved. For instance
the lazy fox
is collected aslal lala ala
. All other visual properties are collected (text color, background color, alignment, etc.). - TextFields: Same as Text
- Other types: no specific data is collected but visual properties are collected.
- Icons: Single characters belonging to the Private Use Area
U+E000..U+F8FF
are collected.
If you believe a particular element might disclose personal data through any of these properties, you must mask it using one of the methods outlined below. A reliable method to assess how a view is rendered in a replay is to navigate to the desired view with the CS SDK active, then use the quick replay link.
Masking example
Section titled Masking exampleHere’s an illustration of a masked view:
Original
-> Replay fully unmasked
-> Replay fully masked
Masking rules priority
Section titled Masking rules priorityThe masking configuration can be set up in two ways:
- Through remote masking configuration in the CSQ Console
- Using the plugin’s public masking APIs
When using SessionReplayMaskingScope
and MaskingConfig
, it’s essential to understand the priority of masking rules in the widget tree. The masking rules specified in a SessionReplayMaskingScope
widget will affect its descendants, and if nested scopes are used, the innermost (closest) scope takes precedence.
Once a rule is triggered, the state is set, and subsequent rules are not applied:
Priority | Rule | Configured via |
---|---|---|
1 | The app or plugin version is fully masked | CSQ Console |
2 | The MaskingConfig object provided to a SessionReplayMaskingScope widget sets the masking rules for its child and descendants. | API |
3 | The MaskingConfig object provided to the ContentsquareRoot or to the SessionReplayMaskingScope widget wrapping the app sets the masking rules for the entire app. | API |
4 | If using manual SDK start The MaskingConfig object provided to the start() method sets the masking rules for the entire app. | API |
5 | Otherwise the widget remains masked except for CustomPaints and SvgImages | default |
Masking rules
Section titled Masking rulesRemote masking configuration
Section titled Remote masking configurationFull Masking
Section titled Full MaskingIn the event of a personal data leak, the full masking configuration in the Console enables a swift and comprehensive masking of the specified application version(s). This ensures that all maskable elements across all screens of these version(s) are completely masked.
This configuration can be accessed through the “Data Masking” tab in the Console and is supported only on CS Flutter plugin version 3.16.0 and later.
It provides three configuration capabilities:
- A toggle to “Mask all eligible versions,” enabling the masking of all app versions embedding the CS Flutter plugin version 3.16.0 or later.
- An “App version” field to mask specific app version(s)
- A “CS SDK version” field to mask the app version(s) embedding the specified plugin version(s)
Public Masking APIs
Section titled Public Masking APIsDefault masking
Section titled Default maskingBy default all images, text and Text fields will be masked. SVGs and CustomPaints are not masked.
Masking/Un-masking in Flutter
Section titled Masking/Un-masking in FlutterSessionReplayMaskingScope
is a Flutter widget that applies specified masking rules to its descendant widgets. This can be used when you want to mask sensitive information during Session Replays, such as user data or private texts.
MaskingConfig
is a class that determines what type of content should be masked during Session Replays. You can customize the masking behavior by setting the masking options for texts, text fields, and images.
How to use SessionReplayMaskingScope
Section titled How to use SessionReplayMaskingScopeTo use SessionReplayMaskingScope
, wrap the desired widget(s) with the SessionReplayMaskingScope
widget and provide a MaskingConfig
object to configure the masking rules.
Here’s an example of how to use SessionReplayMaskingScope
:
SessionReplayMaskingScope( maskingConfig: MaskingConfig.maskAll(), child: const Text('Hello world'),);
In this example, MaskingConfig.maskAll()
is used to mask the Text
widget.
You can also use nested SessionReplayMaskingScope
widgets to apply different masking rules to different parts of your widget tree. Here’s an example:
SessionReplayMaskingScope( maskingConfig: MaskingConfig.maskAll(), child: Column( children: [ const Text('This text will be masked'), SessionReplayMaskingScope( maskingConfig: MaskingConfig(maskTexts: false), child: Text('This text will NOT be masked'), ), ], ),);
In this example, the first Text
widget will be masked, while the second one will not be masked due to the maskTexts: false
option in the nested SessionReplayMaskingScope
.
How to use MaskingConfig
Section titled How to use MaskingConfigMaskingConfig
allows you to configure the masking behavior for different types of content:
maskTexts
: If set totrue
, any widget that creates aRenderParagraph
(example:Text
,RichText
) will be masked.maskTextFields
: If set totrue
, any widget that creates aRenderEditable
(example:TextFormField
,TextField
) will be masked.maskImages
: If set totrue
, any widget that creates an image (example:Image
,BoxDecoration.image
) will be masked.maskSvgImages
: If set totrue
, any widget that creates an SVG image throughflutter_svg
will be masked.maskCustomPaints
: If set totrue
, any custom-made widget that creates aCustomPaint
will be masked. Framework specific decorativeCustomPaint
not containing any sensitive data are not masked.
You can create a custom MaskingConfig
object by using the constructor:
const MaskingConfig({ bool maskTexts, bool maskImages, bool maskTextFields, bool maskSvgImages, bool maskCustomPaints,})
For easier readability and convenience, you can use the following factory constructors:
MaskingConfig.maskAll()
: Returns aMaskingConfig
object with all values set totrue
, meaning everything will be masked.MaskingConfig.unMaskAll()
: Returns aMaskingConfig
object with all values set tofalse
, meaning nothing will be masked.
If any masking parameter is omitted in the MaskingConfig
object, its value is inherited from the nearest SessionMaskingScope
widget in the widget tree.
Advanced features for Experience Monitoring
Section titled Advanced features for Experience MonitoringSend user identifier
Section titled Send user identifierContentsquare provides the ability to search for session(s) associated with a specific visitor, based on an identifier: email, phone number, customer ID…
As these values are typically personal data, from the moment the SDK is collecting the User Identifier
, we immediately encode the value using a hashing algorithm so that the information is hidden and can never be accessed.
Use the following code to send a user identifier:
Contentsquare().sendUserIdentifier('the_neo@one.com');
When called, the native SDK will log:
CSLIB ℹ️ Info: User identifier hashed sent {value}
Sending a user identifier for each session
Section titled Sending a user identifier for each sessionYou may consider sending the user identifier for each session. Although triggering the user identifier at app launch will cover most cases, it may not be adequate in all scenarios. For instance, a session might start when the app is brought to the foreground after being in the background for more than 30 minutes. For further details, refer to the Session definition section. That is why we also recommend sending the user identifier every time the app enters foreground.
Limitations
Section titled Limitations- User identifier max length is 100 characters (if the limit is exceeded, the user identifier will not be handled and you will see an error message in the native Console/Logcat)
- Only the first 15 user identifiers per view will be processed on server side
- The SDK will trim and lowercase user identifier
- User identifier event are not visible in
LogVisualizer
Session Replay Quality
Section titled Session Replay QualityQuality Levels
Section titled Quality LevelsSession Replay is designed by reconstructing the app’s tree structure from selected frames. To minimize performance impact during a session, different quality levels are dynamically applied. Each quality level determines whether certain styles are preserved or omitted while maintaining the overall screen structure and appearance.
High Quality Level
Section titled High Quality LevelAt this level, all supported styles are captured every 200ms, provided a new frame is available. This ensures a comprehensive reproduction of the session’s visual fidelity.
Low Quality Level
Section titled Low Quality LevelThis level captures supported styles only when user interaction ceases, such as during screen scrolling. images are reduced to their average color representation.
Quality Level Adjustment
Section titled Quality Level AdjustmentOur plugin continuously monitors its performance while running. Upon detecting a performance issue, the quality level is automatically reduced to mitigate any potential impact on the application. The quality level may increase when navigating to a new screen, provided the performance issue is resolved.
Quality levels are visibly indicated in the Session Player event stream.
How Session Replay works
Section titled How Session Replay worksInitialization
Section titled InitializationSessions can be collected for Session Replay if the Session Replay feature has been enabled for your project and the session matches the collection criteria.
The following conditions will have an impact on which sessions will be collected:
- User consent: The users have provided their consent (if required)
- Collection rate: The session is designated for collection (see Collection Rate)
- Compatibility: The OS version is supported.
- App version: The app version is not included in the block list (see App Version Block List)
Collection Rate
Section titled Collection RateSession Replay collection is based on a percentage of the total sessions. By default Session Replay collection is disabled.
During the early access phase, the percentage of collected sessions will be set to 1% at the beginning. It will then be adjusted based on:
- The traffic on your app
- The volume of collected sessions set in the contract
Compatibility
Section titled CompatibilitySee the Compatibility matrix.
App Version Block List
Section titled App Version Block ListThe Contentsquare team can include versions of your app in the block list to prevent Session Replay from initiating on these versions. This functionality proves valuable when a problem is identified on a particular version of your app, such as a situation where masking for Personal Data was not implemented. By employing this feature, Session Replay can continue to operate on other app versions, particularly those with necessary fixes implemented.
Collection
Section titled CollectionThe SDK monitors the application lifecycle events and the view hierarchy, capturing Session Replay data based on app behavior, screen content, and user interaction. These events are initially stored locally and later sent to our servers in batches. Subsequently, we aggregate this data to generate actionable visual insights in our Web Application, facilitating your ability to gather insights.
Network and Storage
Section titled Network and StorageBy default, Session Replay data can be sent over cellular network. However, if your app’s context or user preferences necessitate minimal impact on cellular data usage, you can opt to disable data transmission over cellular networks entirely. Once disabled, data will only be sent when the device is connected to Wi-Fi.
To make this adjustment in your project’s configuration, contact your Contentsquare representative.
Storage
Section titled StorageBefore being sent, data is stored in local files on disk up to 30MB on iOS and 20MB on Android. If the limit is reached, Session Replay is halted. It will resume at the next app launch once the SDK can transmit the data.
Requests
Section titled RequestsThe maximum request size is 1 MByte.
Requests are sent:
- Every 10 seconds
- At app hide
- When Replay Link API is called
Android
Section titled AndroidRequests are sent:
- On screen change
- When Replay Link API is called
Performance impact
Section titled Performance impactWe always strive to be non-intrusive, and transparent to the developers of the client app. We apply this rule on the performance as well. These are the technical specifics we can share on performance, if you have any questions feel free to reach out to us.
Performance impact mitigation
Section titled Performance impact mitigationMost operations related to Session Collection are performed on background threads, for the impact on the main thread to be minimal.
We also set up mechanisms that adapts and stop the Session Collection if we detect the feature is using too much memory.
We defined our network strategy to have the lesser impact on CPU and battery of the users devices. We measure these impacts, using Apple Dev Tools, for each release of our SDK.
Session Collection will also stop if we use up to 30Mbytes of local storage.
Performance Benchmarks
Section titled Performance BenchmarksWe have conducted performance tests on the SDK to ensure that it has a minimal impact on the app’s performance. The tests were conducted on a variety of devices and operating systems to ensure that the SDK performs well across different platforms. These tests use the Flutter Gallery ↗ app as a reference and simulate an intensive normal user interaction with the app during 60 seconds (scrolling, tapping, etc.) using the Session Replay feature.
For iPhone 14 with iOS 16.3
Metric | Value |
---|---|
CPU Usage Delta | < 3% |
Memory Usage Delta | < 2% |
Network Usage | < 80kb |
Android
Section titled AndroidFor Pixel 6 with Android 13
Metric | Value |
---|---|
CPU Usage Delta | < 25% |
Memory Usage Delta | < 20% |
Network Usage | < 80kb |
Known limitations
Section titled Known limitations- Images set by
Ink.image
are not currently supported. - Custom shapes are sent as rectangles.
- Blur style is not supported when the app is obfuscated ↗.
- In the event stream of the Session Replay, a tapped element is displayed as
unknown
if it has a transparent parent. - In debug mode, the stability and performance of the Session Replay feature are not guaranteed. Moreover, hot restart and hot reload may introduce unexpected issues. For a reliable and high-performing Session Replay experience, use release or profile builds.
- Masking applied to parent widgets does not apply to the Draggable.feedback ↗ widget. To ensure proper masking while dragging, wrap the Draggable.feedback widget within its own SessionReplayMaskingScope.
Unmaskable Framework specific CustomPaint
classes
Section titled Unmaskable Framework specific CustomPaint classes- CupertinoActivityIndicator
- CupertinoTextSelectionToolbar
- BottomNavigationBar
- DatePickerDialog
- DropdownButton
- InputDecorator
- PhysicalShape
- LinearProgressIndicator
- CircularProgressIndicator
- Stepper
- TabBar
- TextSelectionToolbar
- AnimatedIcon
- GridPaper
- GlowingOverscrollIndicator
- Placeholder