Getting Started
Welcome to the SDK implementation guide!
This guide is designed to help you seamlessly integrate our SDK into your application. By following the outlined steps, you’ll be able to collect and analyze data from your app, within just a few minutes.
Install the SDK
Section titled Install the SDKThe SDK is shipped as a Capacitor plugin which you need to add as a dependency to your Capacitor application.
See Compatibility for more information.
Include the SDK
Section titled Include the SDKInstall the plugin as follows, specifying the exact version you want to install if needed:
npm install @contentsquare/capacitor-pluginnpx cap sync
You do not need to do anything to start the SDK. Now that the SDK is a dependency of your app, it will autostart itself when your application starts.
Validate SDK integration
Section titled Validate SDK integrationStart your application, and check logs for this output:
CSLIB: Contentsquare SDK 6.0.0 starting in app: com.example.testapp
CSLIB ℹ️ Info: Contentsquare SDK v6.0.0 starting in app: com.example.testapp
Check the logs
Section titled Check the logsContentsquare provides logging capabilities that allow you to inspect the raw event data logged by your app in Android Studio, Xcode, or on the Contentsquare platform.
To view all logs, you must enable in-app features: logging is linked to in-app features being enabled or disabled.
Viewing local logs in IDE
Section titled Viewing local logs in IDETo view SDK logs:
- Plug your Android phone into your computer (or use an emulator)
- Open Android Studio and start your app
- Open the
Logcat
view and select your phone or emulator - Filter logs by
CSLIB
-
Unless you are using a simulator, ensure the device you are using is connected to your Mac or is on the same Wi-Fi network.
-
Open the macOS Console app or Xcode.
For the macOS Console app, make sure info messages are included at Choose Action > Include Info Messages ↗.
-
Filter logs by
CSLIB
.
Implement in app-features
Section titled Implement in app-featuresIn-app features are essential for your implementation, as it includes key functionalities like snapshot creation and replay configuration.
To allow Contentsquare users to enable in-app features, perform these tasks:
1. Add the custom URL scheme in your app Info
Section titled 1. Add the custom URL scheme in your app InfoYou have to allow your app to be opened via a custom URL scheme which can be done using one of the following methods:
Xcode
Section titled Xcode- Open your project settings
- Select the app target
- Select the
Info
settings - Scroll to
URL Types
- Set the URL scheme to
cs-$(PRODUCT_BUNDLE_IDENTIFIER)
Text editor
Section titled Text editor-
Open the
Info.plist
of your project -
Add the following snippet:
Info.plist <key>CFBundleURLTypes</key><array><dict><key>CFBundleURLSchemes</key><array><string>cs-$(PRODUCT_BUNDLE_IDENTIFIER)</string></array></dict></array>
2. Call the SDK when the app is launched via a deeplink
Section titled 2. Call the SDK when the app is launched via a deeplinkDepending of the project, there are multiple ways to handle the deeplink opening. Choose the method matching your project structure:
In your AppDelegate
class, complete or implement the function application(app, open url:, options:)
with: Contentsquare.handle(url: url)
In your WindowSceneDelegate
class, you need to:
-
Update
func scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions)
with:if let url = connectionOptions.urlContexts.first?.url {Contentsquare.handle(url: url)} -
Complete or implement
func scene(_ scene: UIScene, openURLContexts URLContexts: Set<UIOpenURLContext>)
with:if let url = URLContexts.first?.url {Contentsquare.handle(url: url)}
In the body
of your main App struct, add the onOpenURL
modifier and call the Contentsquare
SDK to handle the URL:
@mainstruct MyApp: App { var body: some Scene { WindowGroup { MyView() .onOpenURL { url in Contentsquare.handle(url: url) } } }}
Enable in-app features
Section titled Enable in-app featuresTo enable in-app features within your app, you have to first make sure your app is launched in the background. Then, follow the appropriate method described as follows.
On a device: scan the QR code
Section titled On a device: scan the QR codeIn Contentsquare, select the Mobile icon in the menu top bar and scan the QR code with your phone.
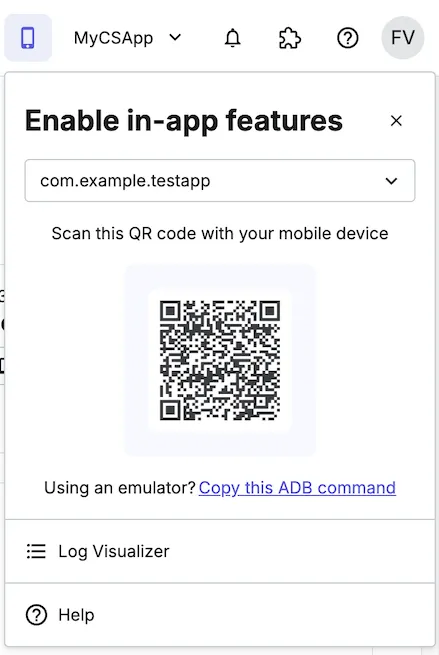
In Contentsquare, select the Mobile icon in the menu top bar and scan the QR code with your phone.
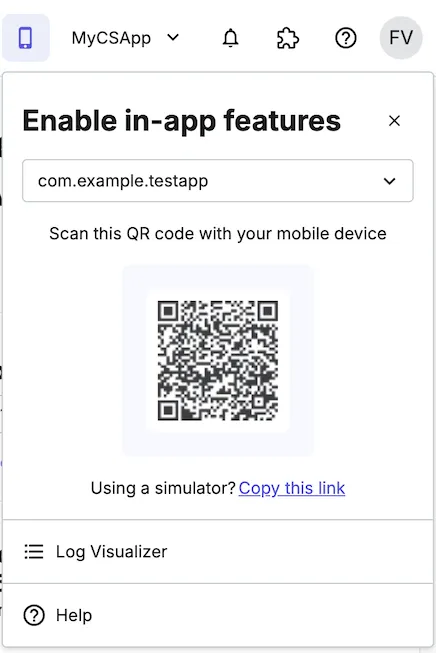
On an emulator/simulator
Section titled On an emulator/simulatorIn Contentsquare, select the Mobile icon in the menu top bar then select your application ID, and “Copy this ADB command”.
In Contentsquare, select the Mobile icon in the menu top bar then select your Bundle ID, and select “Copy this link”. Paste it in Safari on your simulator to trigger the in-app features.
Contentsquare Log Visualizer
Section titled Contentsquare Log VisualizerLog Visualizer is a feature integrated into the Contentsquare SDK. As you navigate and interact with your app, it provides a live view of events detected by the SDK, visible directly on the Contentsquare platform ↗.
- Start your app.
- Select the Mobile icon in the menu top bar then select
Log Visualizer
. - Select the device to inspect.
At this stage, you should see an ‘App start’ or ‘App show’ event being logged.
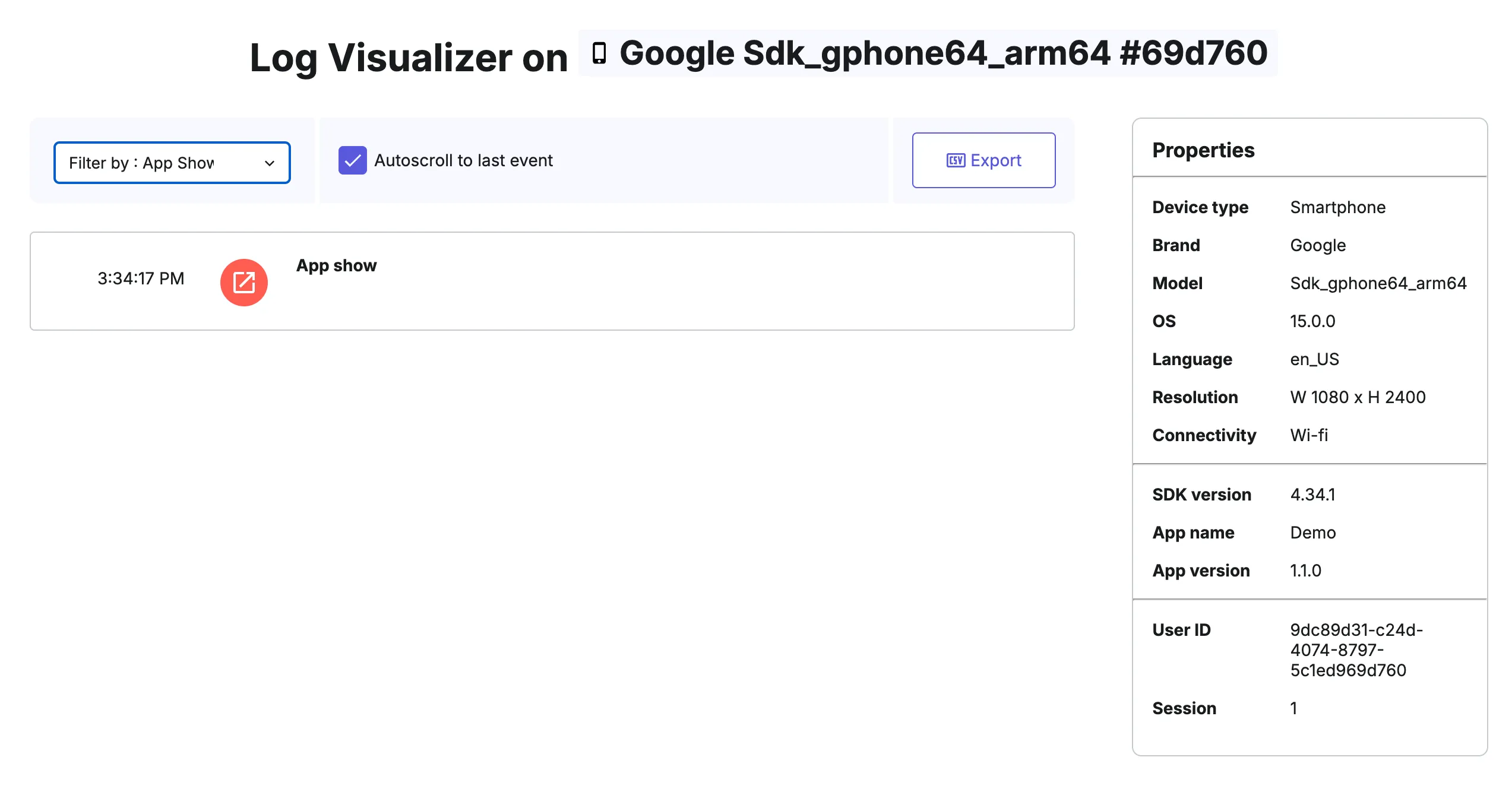
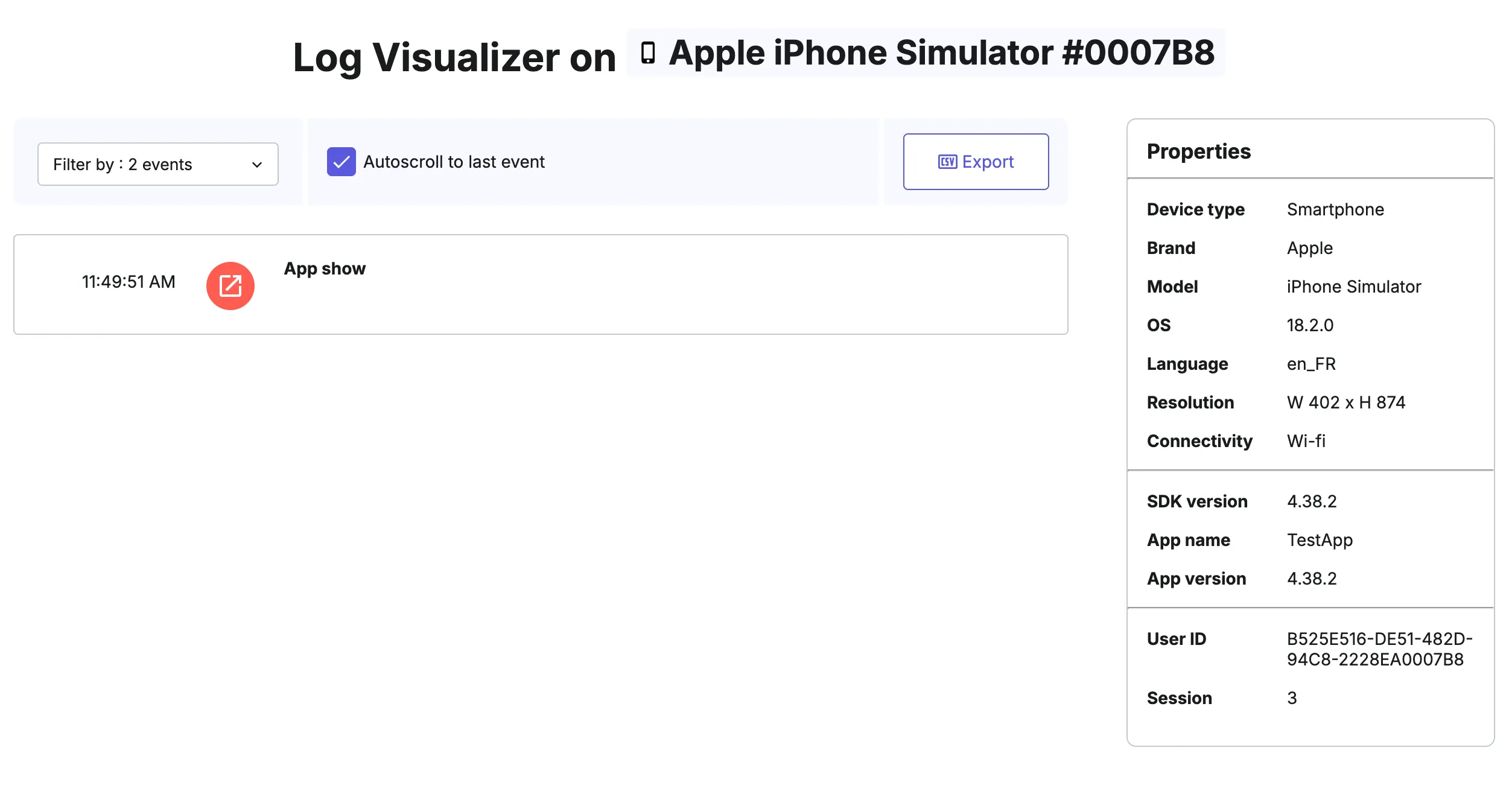
Get user consent
Section titled Get user consentContentsquare collects usage data from your app users. To start tracking, you need your users’ consent for being tracked.
User opt-in
Section titled User opt-inThe SDK treats users as opted-out by default.
Forward user consent with optIn()
. Calling this method generates a user ID and initiates tracking.
import { ContentsquarePlugin } from '@contentsquare/capacitor-plugin';
ContentsquarePlugin.optIn();
Track your first screens
Section titled Track your first screensContentsquare aggregates the user behavior and engagement at the screen level. Start your SDK implementation by tracking key screens like the home screen, product list, product details, or conversion funnel.
Sending screenview events
Section titled Sending screenview eventsScreen tracking is achieved by sending a screenview
event each time a new screen is displayed on the user’s device.
import { ContentsquarePlugin } from '@contentsquare/capacitor-plugin';
ContentsquarePlugin.sendScreenName(screenName) .catch((err) => { // Handle error });
Implementation recommendations
Section titled Implementation recommendationsFrom a functional perspective, a screenview should be triggered in the following cases:
- When the screen appears on the device
- When a modal or pop-up is displayed
- When a modal or pop-up is closed, returning the user to the screen
- When the app is brought back to the foreground (after being minimized)
Screen name handling
Section titled Screen name handlingIt is necessary to provide a name for each screen when calling the screenview API.
As a general rule, keep distinct screen names under 100. As they are used to map your app in Contentsquare, you will want something comprehensive. The screen name length is not limited on the SDK side. However, the limit is 2083 characters on the server side.
More on screen name handling.
Test your setup
Section titled Test your setupTesting your SDK implementation is essential to make sure data is being accurately captured and reported.
To test your setup, simulate user interactions in your app and check that the events are logged correctly in our analytics platform.
You can also use debugging tools such as Android Studio, Xcode, or Log Visualizer to monitor data transmission and ensure everything is running smoothly.
Visualize events in Contentsquare
Section titled Visualize events in ContentsquareUse Log Visualizer to view incoming events within the Contentsquare pipeline. This allows you to monitor the stream in real time.
By simulating user activity, you see incoming screenview and gesture events.
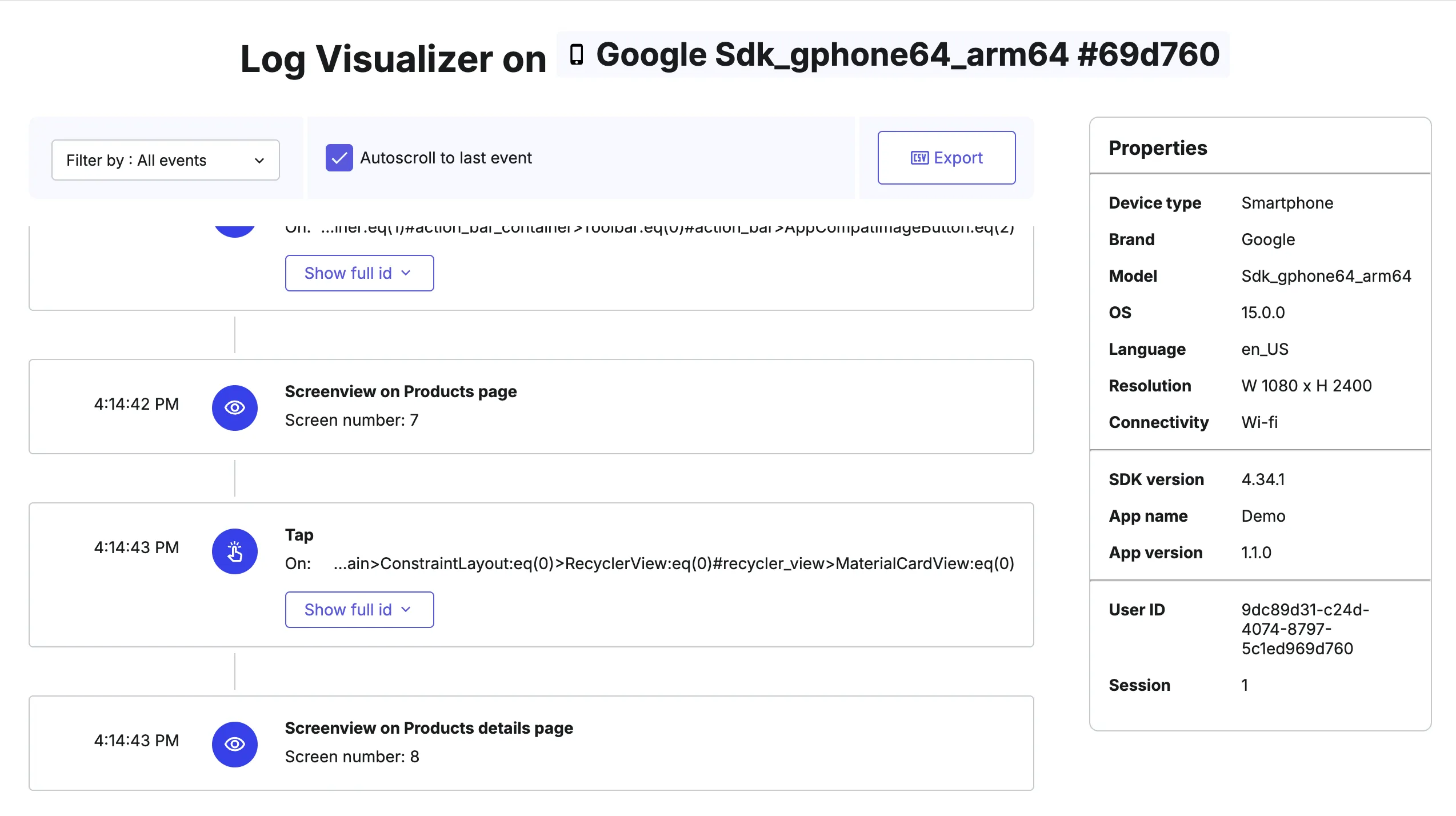
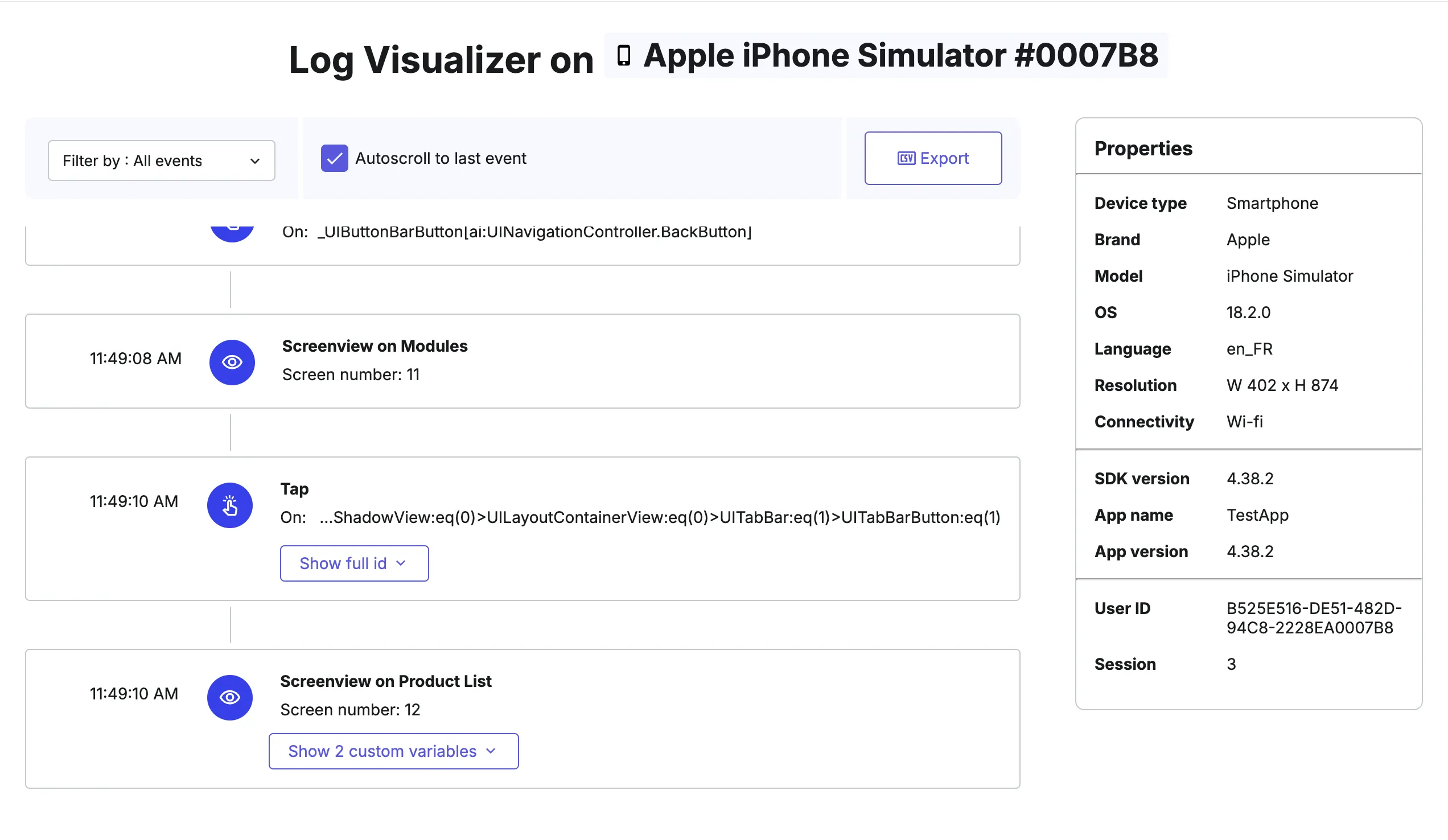
Visualize data in Contentsquare
Section titled Visualize data in ContentsquareIn Journey Analysis
Section titled In Journey AnalysisOpen Journey Analysis ↗ in Contentsquare and visualize the user journeys main steps across your app, screen by screen.
See how to use Journey Analysis on the Help Center ↗.
In Session Replay
Section titled In Session ReplayOpen Session Replay ↗ in Contentsquare and replay the full user session across your app.
See how to use Session Replay on the Help Center ↗
Sample app
Section titled Sample appTo explore some of these features in context, check our Capacitor sample app.
capacitor-sample-app
A sample app giving an example implementation of the Contentsquare SDK
Next steps
Section titled Next stepsWhile screen tracking gives an overview of user navigation, capturing session, screen, or user metadata provides a deeper understanding of the context behind user behavior.
Our SDK offers a wide range of features to enhance your implementation, including Session Replay, Error Monitoring, extended tracking capabilities, and personal data masking.
Proceed with these how-to’s to refine your implementation.