Session Replay
Prerequisites
Section titled PrerequisitesScreen tracking implemented
Section titled Screen tracking implementedAs session data collection will start at the 1st screenview event, it is required to have screen tracking implemented. Make sure to follow the React Native Track screens sections.
Updating to latest SDK version
Section titled Updating to latest SDK versionIn order to enable Session Replay in your app and get the most stable version, it is required to upgrade the SDK to its latest version.
Reminder about User consent
Section titled Reminder about User consentIf you are in the process of implementing the SDK for the 1st time (or choose to take this update as an opportunity to review your Privacy related implementation), make sure to follow the React Native Privacy sections.
WebView
Section titled WebViewWebView events can be collected as part Session Replay under the following conditions:
- The app is a React Native app
- The WebView is registered to the SDK
- The web page implements the Web Tracking Tag
For the full guide to implementation of Webview Tracking, see 📚 Mobile Apps Webview Tracking.
WebView personal data masking is entirely handled on the web side — see 📚 Personal Data handling in WebView.
Testing and Debugging
Section titled Testing and DebuggingEnable Session Replay on your device
Section titled Enable Session Replay on your deviceSince not all sessions are collected (depending on the percentage set in the Contentsquare back office), we have implemented an option to force replay collection on your device for testing and debugging purposes. This option can be enabled from the in-app features settings:
- Enable in-app features
- Open in-app features settings with a long press on the snapshot button
- Under “Session Replay”, toggle “Enable Session Replay” on
- Kill the app
- Start app, a new session is starting with Session Replay enabled
How do I know if Session Replay is enabled?
Section titled How do I know if Session Replay is enabled?There are 2 places where you can check if Session Replay is enabled:
In the logs: The log I/CSLIB|SessionReplay: Starting Session Replay.
will confirm that Session Replay is enabled.
In in-app features settings: Below the “Enable “will start at next app start” (see below Access the replay), you will either see:
No replay link available
which means Session Replay is not running for the current sessionGet Replay link
which means Session Replay is running for the current session
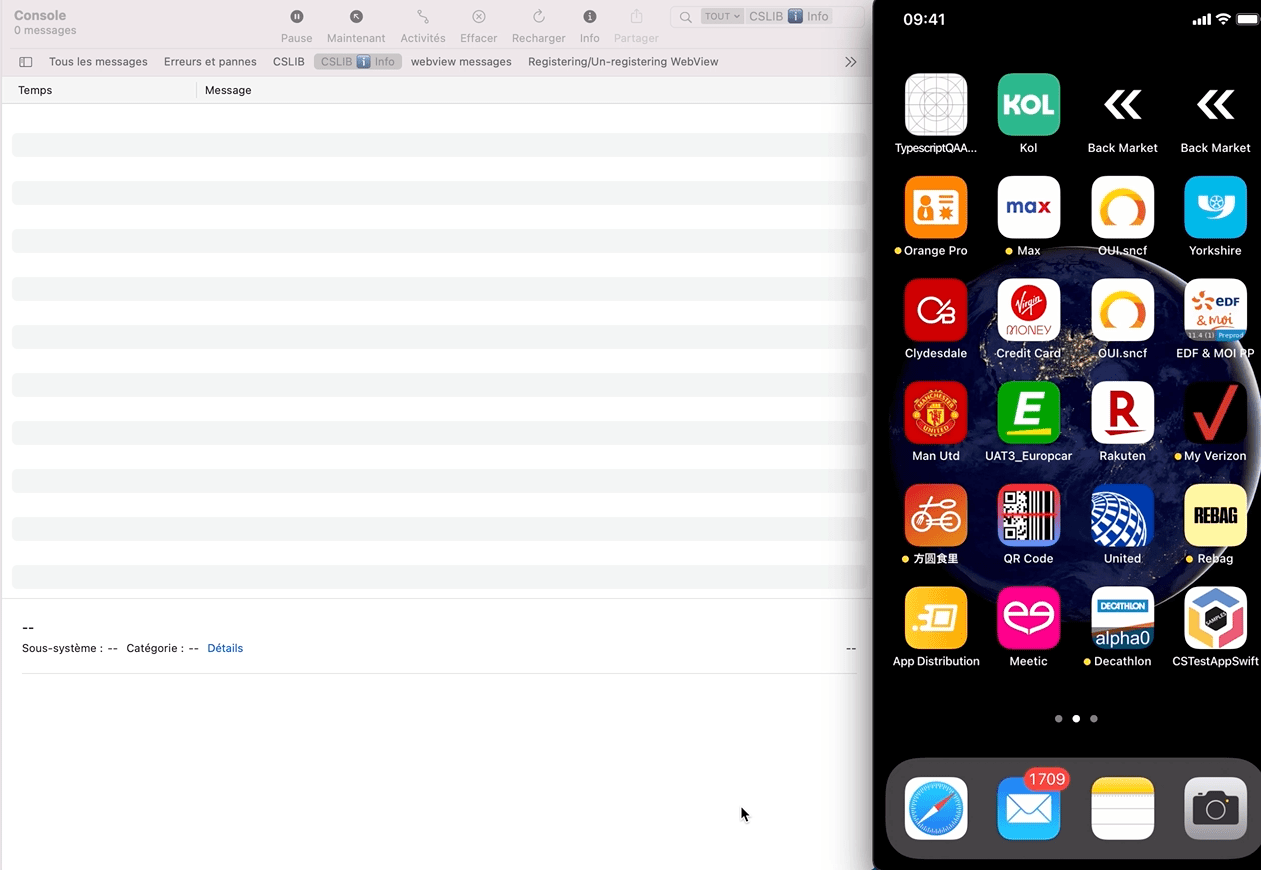
There are 2 places where you can check if Session Replay is enabled:
In the logs: The log Session Recording is starting.
will confirm that Session Replay is enabled.
In in-app features settings: Below the “Enable “will start at next app start” (see below Access the replay), you will either see:
No replay link available
which means Session Replay is not running for the current sessionGet Replay link
which means Session Replay is running for the current session
Access the replay
Section titled Access the replayThe replay can be accessed by tapping on Get replay link
button from the in-app features settings:
The replay will be available within 5 minutes. Only the “ended screen views” are processed (we know a screenview is ended when we start receiving data for the next screenview). This means that you will be able to replay your session up to the previous screenview if the session is still running.
The session can be accessed by tapping on Get replay link
button from the in-app features settings:
The replay will be available within 5 minutes. Only the “ended screen views” are processed (we know a screenview is ended when we start receiving data for the next screenview). This means that you will be able to replay your session up to the previous screenview if the session is still running.
Provide custom fonts (iOS only)
Section titled Provide custom fonts (iOS only)It is required to send us the custom fonts you use in your app so we can use them to render text properly.
Upload the fonts in otf
, ttf
, woff
or woff2
format directly from the player as described in Managing iOS Fonts In The Player ↗ on the Help Center.
Personal Data Masking
Section titled Personal Data MaskingThe Session Replay feature collects every user interaction within your app. In order to respect the user’s right to privacy, the Contentsquare SDK:
- Masks everything by default
- Allows you to control which part of the user interface is collected via our masking and un-masking component.
Masking mechanisms
Section titled Masking mechanismsAlthough the implementation is done once for both iOS and Android on React Native, the visual appearance of the masking itself will be different for each platform:
Every single UI element is converted into a highly pixelated image to reach a very low resolution. Text and images will appear very blurry so that the content cannot be identified.
Original VS Replay fully masked
Section titled Original VS Replay fully masked- Images are not collected, a placeholder is sent instead of the content; the “IMG” placeholder will be displayed in the frame of the element.
- Text: text is replaced by “la” repeated as many times as needed to equal the original character count. White characters are preserved. For instance
the lazy fox
is collected aslal lala ala
. All other visual properties are collected (text color, background color, alignment, etc.). - TextFields: same as Text
- For all other types: no specific data is collected but visual properties are collected. If you think a specific element can reveal personal data from one of these properties you have to mask it using one of the methods presented below. A good way to check how a view is rendered in replays is to navigate to the desired view with the CS SDK running then use the quick replay link.
Original VS Replay fully masked
Section titled Original VS Replay fully maskedMasking rules priority
Section titled Masking rules priorityGeneral case
Section titled General caseThe SDK determines whether a view is masked by evaluating the rules in the order specified below. Once a rule is triggered, the state is set, and subsequent rules are not applied:
Priority | Rule | Configured via |
---|---|---|
1 | The app or SDK version is fully masked | CSQ Console |
2 | A component is specifically masked or unmasked | API |
3 | A parent is specifically masked or unmasked | API |
4 | Otherwise the default masking state is applied | API |
Masking rules
Section titled Masking rulesThe masking rules can be applied in two ways:
- Through remote masking configuration in the CSQ Console
- Using the SDK public masking APIs
Remote masking configuration in the CSQ Console
Section titled Remote masking configuration in the CSQ ConsoleFull masking
Section titled Full maskingIn the event of a personal data leak, the full masking configuration in the Console enables a swift and comprehensive masking of the specified application version(s). This ensures that all elements across all screens of these version(s) are completely masked.
This configuration can be accessed through the “Data Masking” tab in the Console and is supported only on Contentsquare React Native Bridge 4.13.0 and later.
It provides three configuration capabilities:
- A toggle to “Mask all eligible versions,” enabling the masking of all app versions embedding the Contentsquare React Native Bridge 4.13.0 or later.
- An “App version” field to mask specific app version(s)
- A “CS SDK version” field to mask the app version(s) embedding the specified SDK versions for iOS and Android projects, respectively. This means that instead of providing the Contentsquare React Native Bridge version, one should specify the iOS or Android SDK version(s) listed in the changelog.
Public masking APIs
Section titled Public masking APIsDefault masking
Section titled Default maskingAll components are initially masked by default. To modify this default masking state, utilize the setDefaultMasking
API, preferably within a useEffect
in the root file (such as App.js
). This ensures proper initialization and handling when the component mounts.
If you choose to unmask the entire app using the setDefaultMasking
API, be aware that you will need to individually mask all personal data within the app. Additionally, ensure that you consistently mask personal data on new screens in the future, if necessary.
/*** Set the default masking of all the views.* @param isMasking true mask all views by default.* false unmask all views by default.* The default value is true.*/setDefaultMasking(boolean isMasking)
Masking/Unmasking Specific Component
Section titled Masking/Unmasking Specific ComponentTo mask or unmask specific views or components you can use the <CSMask isMasking={boolean}>
component. Control masking by setting the isMasking
prop:
isMasking={true}
(default, can be omitted): Masks all children components.isMasking={false}
: Unmasks all children components.
In this example below, all children views inside <CSMask>
will follow the rule of the closest parent prop isMasking
.
// Masks or unmasks all child components based on isMasking prop<CSMask isMasking={true | false}> <View> <Text style={styles.title}>Buy my amazing merch!</Text> <Text> Hurry up! This offer won't be available tomorrow. Only $99.98 to get your hands on a wonderful, brand-new, life-changing item. </Text> </View></CSMask>
Masking and Unmasking behaviors on a parent view
Section titled Masking and Unmasking behaviors on a parent viewThe masking state of a parent view propagates to all nested views unless explicitly overridden by a nested <CSMask>
component. This enables flexible masking and unmasking behaviors within complex component structures.
// Masks or unmasks all child components based on isMasking prop<CSMask isMasking={true}> <View> <Text>This content is masked.</Text>
<CSMask isMasking={false}> <Text>This specific content is unmasked, despite the parent mask.</Text> </CSMask> </View></CSMask>
In this example, the inner <CSMask>
explicitly overrides the parent view’s masking behavior.
Implementation recommendations
Section titled Implementation recommendationsAvoid wrapping the Root Navigator
Section titled Avoid wrapping the Root Navigator<CSMask>
should not wrap the Root Navigator it could cause unwanted results. If you want to set the default masking behavior for the whole application you should use setDefaultMasking(boolean isMasking)
. <CSMask>
should be used at component level.
Avoid dynamically changing isMasking
prop
Section titled Avoid dynamically changing isMasking propWhile <CSMask>
supports toggling masking at the instance level, we do not recommend changing the isMasking
prop dynamically based on conditions like this:
<CSMask isMasking={someCondition ? true : false}>...</CSMask>
This approach may lead to unexpected behavior, such as personal data leaks. Instead, structure your layout in a way that ensures predictable masking behavior without frequently modifying the isMasking
prop dynamically.
Keeping track of what is masked
Section titled Keeping track of what is maskedThe SDK doesn’t provide a list of what is currently masked. If you need to keep track of it, you probably will have to write your specific wrapper.
Advanced features for Experience Monitoring
Section titled Advanced features for Experience MonitoringSend user identifier
Section titled Send user identifierContentsquare provides the ability to search for session(s) associated with a specific visitor, based on an identifier: email, phone number, customer ID… As these values are typically personal data, from the moment the SDK is collecting the User Identifier, we immediately encode the value using a hashing algorithm so that the information is hidden and can never be accessed.
Use the following code to send a user identifier:
import Contentsquare from "@contentsquare/react-native-bridge";Contentsquare.sendUserIdentifier("any_identifier");
When called, the SDK will log:
I/CSLIB: User identifier hashed sent {value}
CSLIB ℹ️ Info: User identifier hashed sent {value}
Sending a user identifier for each session
Section titled Sending a user identifier for each sessionYou may want to send the user identifier for each session. While triggering the user identifier at app launch will cover most cases, it will not be enough. A session can start when the app is put in foreground, after staying in background for more than 30 minutes. See Session definition section for more information.
That is why we also recommend sending the user identifier every time the app enters foreground.
You can use AppState
API ↗ to detect foreground and trigger a sendUserIdentifier()
.
Limitations
Section titled Limitations- User identifier max length is 100 characters (if the limit is exceeded, the user identifier will not be handled and you will see an error message in the Console/Logcat)
- Only the first 15 user identifiers per view will be processed on server side
- The SDK will trim and lowercase user identifier
- User identifier event are not visible in Log visualizer
Integrations
Section titled IntegrationsContentsquare provides the ability to retrieve the link of the replay to be attached to other vendors such as Voice of Customer or Crash reporting tools.
Subscribe to Session Replay Link changes
Section titled Subscribe to Session Replay Link changesUse onSessionReplayLinkChange
to subscribe to Session Replay link updates (1). Then use the returned subscriber to remove the subscription when is not needed anymore. (2)
useEffect(() => { const subscriber = Contentsquare.onSessionReplayLinkChange((link) => { // 1 currentSessionReplaylink = link; });
return () => { if (subscriber) { // 2 subscriber.remove(); } };}, []);
I/CSLIB: SessionReplay link: https://app.contentsquare.com/quick-playback/index.html?pid={projectId}&uu={userId}&sn={sessionNumber}&recordingType=cs
When the callback is called, the SDK will log:
If you have a Contentsquare account, you can use this link to directly watch your current Session Replay on the Contentsquare Platform.
The replay will be available within 5 minutes. Only the “ended screen views” are processed (we know a screenview is ended when we start receiving data for the next screenview). This means that you will be able to replay the session up to the previous screenview if the session is still running.
Get current replay Link
Section titled Get current replay Linkconst link = await Contentsquare.getCurrentSessionReplayLink();
How Session Replay works
Section titled How Session Replay worksInitialization
Section titled InitializationSessions can be collected for Session Replay if the Session Replay feature has been enabled for your project and the session matches the collection criteria.
The following conditions will have an impact on which sessions will be collected:
- User consent: The users have given their consent (if required)
- Collection rate: The session is being drawn for collection (see Collection Rate below)
- Compatibility: The OS version is supported.
- App version: The app version is not part of the block list (see App version block list below)
Note that, when Session Replay is turned off, no content specific to Session Replay is collected whatsoever. Also note that the standard Contentsquare analytics tracking remains unaffected by this.
Collecting rate
Section titled Collecting rateSession Replay collection is based on a percentage of the total sessions. By default Session Replay collection is disabled.
During the early access phase, the percentage of collected sessions will be set to 1% at the beginning. It will then be adjusted according to:
- The traffic on your app
- The volume of collected sessions set in the contract
Compatibility
Section titled CompatibilitySee compatibility.
App version block list
Section titled App version block listThe Contentsquare team can add versions of your app in the block list to make sure Session Replay does not start on these versions. This is done when a problem is discovered on a specific version of your app, such as a Personal Data for which the masking was forgotten. This allows to keep Session Replay to work on the other app versions (especially the new ones with the fix).
Collection
Section titled CollectionThe SDK monitors the application lifecycle events and the view hierarchy, and generates Session Replay data from the behavior of the app, the content of the screen and the interaction of the user. These events are then locally stored, and eventually sent to our servers in batches. We then aggregate that data to create usable visual information into our Web Application, which you use to gather insights.
Network and Storage
Section titled Network and StorageBy default, Session Replay data can be sent over cellular network. If the particular context of your app or users requires a very limited impact on cellular data consumption, sending data over cellular network can be completely disabled. Once disabled, data will be sent when the device is using Wi-Fi.
Reach out to your Contentsquare contact that will make the adjustment in your project’s configuration.
Storage
Section titled StorageBefore being sent, data is stored in local files on disk up to 30MB on iOS and 20MB on Android. If the limit is reached, Session Replay is stopped. It will restart at the next app launch, once the SDK has been able to send the data.
Requests
Section titled RequestsThe maximum request size is 1Mbyte.
Android
Requests are sent:
- Every 5 seconds
- On screen change
- When Replay Link API is called
iOS
Requests are sent:
- Every 10 seconds
- At app hide
- When Replay Link API is called
Performance impact
Section titled Performance impactAndroid
Section titled AndroidPerformance impact mitigation
Section titled Performance impact mitigationMost operations related to Session Replay are performed on dedicated background threads, for a minimal impact on the main thread. If CPU usage is too high for background tasks, some expensive calculations will be discarded. To make sure of this, we run performance tests on testing applications, along with the Android profiler.
If too much time is spent on the main thread, the quality level will decrease automatically: from high to medium to low to a complete stop if required. Once conditions are back to normal, quality level will be changed back to the default value set in the configuration.
We defined our network strategy to have the lesser impact on CPU, memory and data consumption of the users devices. We measure these impacts, using the Android profiler and dedicated logging system, for each release of our SDK.
You can reduce the quality level if you want to favor performance impact over quality.
Performance test results
Section titled Performance test resultsWe always strive to be non-intrusive, and transparent to the developers of the client app. We apply this rule on the performance as well. These are the technical specifics we can share on performance, if you have any questions feel free to reach out to us.
The performance results were obtained under the following conditions:
Condition | Value |
---|---|
Device model | Pixel 5 |
Android version | 33 (Android 13) |
Quality level | High |
Default Masking State | Disabled |
Property | Value |
---|---|
Total % on main thread (CPU Profiling) | < 10 % |
Memory overhead | 10 MB |
Session Replay data transmitted over network during 1 minute of use: | |
- with “High quality” level | < 300 kB |
- with “Medium quality” level | < 200 kB |
- with “Low quality” level | < 100 kB |
Additional info on performance test procedure
Section titled Additional info on performance test procedureCPU Usage Test We are profiling our Demo app with and without the SDK dependency by following the same use case. We are measuring the CPU peak for both versions of the app and we are computing the difference.
RAM Usage Test We are profiling our Demo app with and without the SDK dependency by following the same use case. We are measuring the RAM usage peak for both versions of the app and we are computing the difference.
Memory Leak Test We are profiling our demo app and we are using the ADB Monkey tool to create random user input events. During this time we are monitoring the RAM behavior for any anomaly. We are using the Memory Profiler to identify leaks.
Data consumption We replay a predefined, repeatable and automated user scenario on our demo app and all data sent is measured in kB/min.
JankStats If you use the alpha library from Google to monitor the jank frames and you already have some jank frames in your application (main thread overwhelmed), you can expect an increase of 1% or 2% with Session Replay activated. Session Replay requires to perform tasks on the main thread (screen capture and view hierarchy inspection).
We always strive to be non-intrusive, and transparent to the developers of the client app. We apply this rule on the performance as well. These are the technical specifics we can share on performance, if you have any questions feel free to reach out to us.
Performance impact mitigation
Section titled Performance impact mitigationMost operations related to Session Replay are performed on background threads, for the impact on the main thread to be minimal. To make sure of this, we run performance tests on testing applications, using XCTMetric and Hitch Time Ratio measures.
We also set up mechanisms that stop Session Replay if we detect the feature is using too much memory.
We defined our network strategy to have the lesser impact on CPU and battery of the users devices. We measure these impacts, using Apple Dev Tools, for each release of our SDK.
Session Replay will also stop if we use up to 30Mbytes of local storage.
You can reduce the quality level if you want to favor performance impact over quality.
Performance test results
Section titled Performance test resultsThe following performance results were obtained under the following conditions:
Condition | Value |
---|---|
Device model | iPhone 7 |
iOS version | 15.7.8 |
Test App built using Xcode version | 14.3.1 |
Test App built with Swift version | 5.8 |
Quality level | High |
Default Masking State | Disabled |
We conducted the tests using a default Master-Detail
app built using AdHoc distribution with no app thinning and with Swift symbols stripped. In the app, the SDK was making calls to the Public APIs, running and collecting data in its default state.
Property | Value |
---|---|
Max CPU overhead | <10% |
Max RAM usage | <5MB |
Session Replay data transmitted over network during 1 minute of use | |
- with “High quality” level | <2Mbytes |
- with “Medium quality” level | <1Mbytes |
- with “Low quality” level | <900Kbytes |
Known limitations
Section titled Known limitationsDiscrepancy of the default iOS Font
Section titled Discrepancy of the default iOS FontThere is a discrepancy of the default iOS Font between devices and Session Replay.
Troubleshooting
Section titled TroubleshootingRequests are not sent from an Android emulator / iOS simulator
Section titled Requests are not sent from an Android emulator / iOS simulatorIf you struggle to watch a replay collection on an emulator/simulator, it may be due to some network constraints applied on your computer (VPN, company network restrictions, etc.). Check your configuration or use a real device.
See following sections for more information about our endpoints and requests:
Very long session on an Android emulator / iOS simulator
Section titled Very long session on an Android emulator / iOS simulatorIt is important to remember that an app kill does not end a session. See Session definition for more information. If you leave the simulator/emulator running with the app in foreground, the session will not end, even if you are inactive. To better reflect actual end user behavior and avoid unusually long sessions (last hours), put the app in background or kill it.
Elements being unmasked when scrolling on Android
Section titled Elements being unmasked when scrolling on AndroidIf you encounter an issue where elements positioned above a ScrollView appear unmasked when scrolling, it may be due to the ScrollView clipping behavior. To resolve this, add the removeClippedSubviews={true}
property to the ScrollView. This will ensure that only the visible subviews are rendered, helping prevent masking issues.
<View> <Image source={{ uri: image }} /> <ScrollView removeClippedSubviews={true}> {items.map(item => ( <ListItem key={item.id} item={item} /> ))} </ScrollView><View>